Add Request Parameters
By default, the AppSignal integrations track request parameters for web requests in supported libraries. These include query parameters and the POST request body*. For background jobs, we store the job arguments in the parameters.
You can set custom parameters on a transaction or span. Modifying the parameters of a transaction will overwrite the data set by the AppSignal instrumentations.
All parameters are filtered by our integrations before being sent to our servers.
See the table below for a list of accepted root values per language. Each nested object can contain values that result in valid JSON (strings, integers, floats, booleans, nulls, etc.).
Language | Accepted root values |
---|---|
Ruby | Arrays, Hashes |
JavaScript | Arrays, Objects |
Elixir | Lists, Maps |
Python | Lists, Maps |
Go | JSON serialized string |
Java | JSON serialized string |
PHP | JSON serialized string |
The below code sample shows how to set custom request parameters:
# Values are merged at the top level Appsignal.add_params( :post => { :title => "My title", :body => "Post text." } ) Appsignal.add_params(:user => { :login => "some_name" }) Appsignal.add_params(:user => { :id => 123 }) # Parameters: # { # :post => { :title => "My title", :body => "Post text." }, # :user => { :id => 123 } # }
Appsignal.Span.set_sample_data( Appsignal.Tracer.root_span, "params", %{ post: %{ title: "My new blog post!", body: "Some long blog post text." } } )
import { setParams } from "@appsignal/nodejs"; setParams({ post: { title: "My new blog post!", body: "Some long blog post text.", }, });
from appsignal import set_params set_params({ post: { title: "My new blog post!", body: "Some long blog post text." } })
// Additional setup is required to first fetch or create a new span import ( "encoding/json" "go.opentelemetry.io/otel/attribute" "go.opentelemetry.io/otel/trace" ) params := map[string]interface{}{ "param1": "value1", "param2": "value2", "nested": map[string]interface{}{ "param3": "value3", "param4": "value4", }, } json, _ := json.Marshal(params) span.SetAttributes(attribute.String("appsignal.request.query_parameters", string(json)))
// Additional setup is required to first fetch or create a new span import io.opentelemetry.api.trace.Span; // We're using the Jackson JSON library // You may need to add it to your project as a dependency import com.fasterxml.jackson.databind.ObjectMapper; import java.util.HashMap; import java.util.Map; Map<String, Object> queryParams = new HashMap<>(); queryParams.put("param1", "value1"); queryParams.put("param2", "value2"); Map<String, String> nested = new HashMap<>(); nested.put("param3", "value3"); nested.put("param4", "value4"); queryParams.put("nested", nested); try { ObjectMapper mapper = new ObjectMapper(); String jsonString = mapper.writeValueAsString(queryParams); Span span = Span.current(); span.setAttribute("appsignal.request.query_parameters", jsonString); } catch (Exception e) { // Add error handling here }
<?php // Additional setup may be required to first fetch or create a new span use OpenTelemetry\API\Trace\Span; $params = [ 'param1' => 'value1', 'param2' => 'value2', 'nested' => [ 'param3' => 'value3', 'param4' => 'value4', ], ]; $json = json_encode($params); $span = Span::getCurrent(); $span->setAttribute( 'appsignal.request.query_parameters', $json );
For certain languages, additional setup is required. Please follow the instructions for these languages:
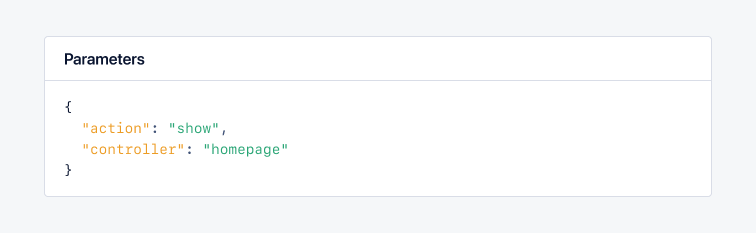
Request payload data
The languages supported through our OpenTelemetry beta store the request query parameters and request payload data separately. This section describes how to set the request payload data from POST requests.
You can set custom request payload data on a span. Modifying the request payload data of a span will overwrite the data set by the AppSignal instrumentations.
All parameters are filtered by our integrations before being sent to our servers.
See the table below for a list of accepted root values per language. Each nested object can contain values that result in valid JSON (strings, integers, floats, booleans, nulls, etc.).
Language | Accepted root values |
---|---|
Go | JSON serialized string |
Java | JSON serialized string |
PHP | JSON serialized string |
The below code sample shows how to set custom request payload data:
// Additional setup is required to first fetch or create a new span import ( "encoding/json" "go.opentelemetry.io/otel/attribute" "go.opentelemetry.io/otel/trace" ) payload := map[string]interface{}{ "param1": "value1", "param2": "value2", "nested": map[string]interface{}{ "param3": "value3", "param4": "value4", }, } json, _ := json.Marshal(payload) span.SetAttributes(attribute.String("appsignal.request.payload", string(json)))
// Additional setup is required to first fetch or create a new span import io.opentelemetry.api.trace.Span; // We're using the Jackson JSON library // You may need to add it to your project as a dependency import com.fasterxml.jackson.databind.ObjectMapper; import java.util.HashMap; import java.util.Map; Map<String, Object> payload = new HashMap<>(); payload.put("param1", "value1"); payload.put("param2", "value2"); Map<String, String> nested = new HashMap<>(); nested.put("param3", "value3"); nested.put("param4", "value4"); payload.put("nested", nested); try { ObjectMapper mapper = new ObjectMapper(); String jsonString = mapper.writeValueAsString(payload); Span span = Span.current(); span.setAttribute("appsignal.request.payload", jsonString); } catch (Exception e) { // Add error handling here }
<?php use OpenTelemetry\API\Trace\Span; $params = [ 'param1' => 'value1', 'param2' => 'value2', 'nested' => [ 'param3' => 'value3', 'param4' => 'value4', ], ]; $json = json_encode($params); // Additional setup may be required to first create a new span $span = Span::getCurrent(); $span->setAttribute( 'appsignal.request.payload', $json );
For certain languages, additional setup is required. Please follow the instructions for these languages:
Limitations
If the application sets request query parameters or request payload data multiple times, the Ruby gem will merge values at the root level. For other integrations, only the last set value is stored.