Adding Tags to a Request
You can use tagging to supply extra context on errors and performance incidents. Tagging can help to add information that is not already part of a request, such as the session data, headers, environment data, or parameters. Tags can also be used to filter on samples within an incident to find all errors for a specific user or slow pages for a particular locale.
You can set tags wherever the current transaction or span is accessible. We recommend calling it before your application code runs in a request, such as a callback. You can see what tags apply to a sample on the Error and Performance incident page.
Using Link Templates it's possible to add links to your application with tag data, such as the admin panel for the signed-in user. You can see this in practice in the below screenshot, with the Link 1
and Link 2
tags.
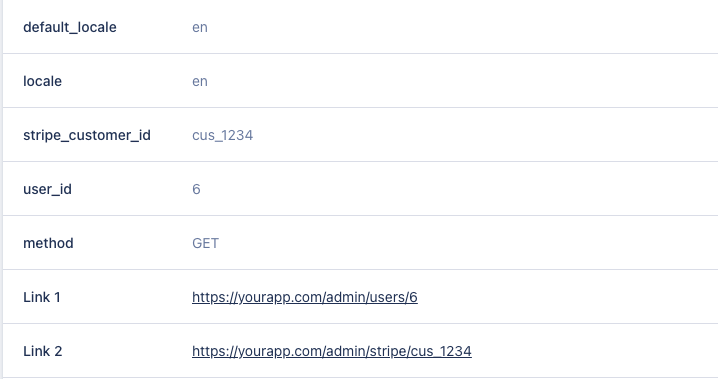
Ruby
With the AppSignal for Ruby gem, use the Appsignal.add_tags
helper methods to add tags to error and performance samples. On Ruby gem version 3 and older, use the Appsignal.set_tags
helper.
You can use Appsignal.add_tags
wherever an active transaction is accessible, we recommend calling it before your application code runs in the request, such as in a before_action
using Rails.
before_action do Appsignal.add_tags( :user_id => current_user.id, :stripe_customer_id => stripe_customer_id, :locale => I18n.locale, :default_locale => I18n.default_locale ) end
Limitations
Tags that do not meet these limitations are dropped without warning.
- The tag key must be a String or Symbol.
- The tagged value must be a String, Symbol, Integer or Boolean (true/false).
- Boolean values are supported in Ruby gem 3.11 and newer.
- The length of the tag value must be less than 256 characters.
- Nested hash values are not supported for tags, please use custom data instead.
# Unsupported: hash value type is not supported Appsignal.add_tags( :i18n => { :locale => I18n.locale, :default_locale => I18n.default_locale } )
Elixir
Use the Appsignal.Span.set_sample_data
function to supply extra context on errors and performance samples. Use the "tags"
sample key for the function to add tags to the span.
Appsignal.Span.set_sample_data( Appsignal.Tracer.root_span, "tags", %{ locale: "en", user_id: user_id, stripe_customer_id: stripe_customer_id, locale: locale, default_locale: default_locale } )
Last call is leading
The set_sample_data
helper can be called multiple times, but only the last value will be retained. When the code is run below:
Appsignal.Span.set_sample_data(Appsignal.Tracer.root_span, "tags", %{locale: "en"}) Appsignal.Span.set_sample_data(Appsignal.Tracer.root_span, "tags", %{user: "bob"}) Appsignal.Span.set_sample_data(Appsignal.Tracer.root_span, "tags", %{locale: "de"})
it will result in the following tags being stored:
%{ locale: "de" }
Setting sample data on a span
To add sample data to a span as soon as it's created, add a custom_on_create_fun
:
defmodule MyApp.Appsignal do def custom_on_create_fun(_span) do Appsignal.Span.set_sample_data(Appsignal.Tracer.root_span, "tags", %{"locale": "en"}) end end
Then, add it to your app's configuration:
# config/config.exs config :appsignal, custom_on_create_fun: &MyApp.Appsignal.custom_on_create_fun/1
The custom_on_create_fun
requires AppSignal for Elixir version 2.8.3 or higher.
Limitations
Tags that do not meet these limitations are dropped without warning.
- The tag key must be a
String
orAtom
. - The tagged value must be a
String
,Atom
orInteger
. - The length of the tag value must be less than 256 characters.
- Nested map values are not supported for tags, please use custom data instead.
# Unsupported: map value type is not supported Appsignal.Span.set_sample_data( Appsignal.Tracer.root_span, "tags", %{ i18n: %{ locale: "en_GB", default_locale: "en_US" } } )
Node.js
Import the setTag
helper function to add tags to spans for errors and performance samples.
import { setTag } from "@appsignal/nodejs"; setTag("user_id", user_id); setTag("stripe_customer_id", stripe_customer_id); setTag("locale", locale); setTag("default_locale", default_locale);
Limitations
Tags that do not meet these limitations are dropped without warning.
- The tag key must be a
String
. - The tagged value must be a
String
,Number
orBoolean
. - The length of the tag value must be less than 256 characters.
- Nested object values are not supported for tags, please use the
setCustomData
helper function instead.
const tracer = appsignal.tracer(); const span = tracer.currentSpan(); # Unsupported: object value type is not supported span.set("i18n", { locale: "en_GB", default_locale: "en_US" });
Python
Import the set_tag
helper method to add tags to spans for errors and performance samples.
from appsignal import set_tag set_tag("user_id", user_id) set_tag("stripe_customer_id", stripe_customer_id) set_tag("locale", locale) set_tag("default_locale", default_locale)
Limitations
Tags that do not meet these limitations are dropped without warning.
- The tag key must be a String.
- The tagged value must be a String, Integer, Float or Boolean.
- The length of the tag value must be less than 256 characters.
- Nested object values are not supported for tags, please use the
set_custom_data
helper function instead.
Go
In a Go app, to add an AppSignal tag to a span on a trace, first fetch the active span or create a new span as described on our Go custom instrumentation page.
Then, set an attribute on the span using the attribute helper that matches the type of the tag value, see also the tag limitations below.
The span attribute name has the following format: appsignal.tag.<tag name>
. Replace <tag name>
with the name of the tag.
// Example format span.SetAttributes(attribute.String("appsignal.tag.<tag_name>", "string")) // Supported types span.SetAttributes(attribute.String("appsignal.tag.my_tag_string", "tag value")) span.SetAttributes(attribute.StringSlice("appsignal.tag.my_tag_int64", []string{"abc", "def"})) span.SetAttributes(attribute.Bool("appsignal.tag.my_tag_bool_true", true)) span.SetAttributes(attribute.Bool("appsignal.tag.my_tag_bool_false", false)) span.SetAttributes(attribute.BoolSlice("appsignal.tag.my_tag_bool_slice", []bool{true, false})) span.SetAttributes(attribute.Float64("appsignal.tag.my_tag_float64", 12.34)) span.SetAttributes(attribute.Float64Slice("appsignal.tag.my_tag_float64_slice", []float64{12.34, 56.78})) span.SetAttributes(attribute.Int("appsignal.tag.my_tag_int", 1234)) span.SetAttributes(attribute.IntSlice("appsignal.tag.my_tag_int", []int{1234, 5678})) span.SetAttributes(attribute.Int64("appsignal.tag.my_tag_int64", 1234)) span.SetAttributes(attribute.Int64Slice("appsignal.tag.my_tag_int64_slice", []int64{1234, 5678}))
Limitations
Tags that do not meet these limitations are dropped without warning.
- The tag key must be a String.
- The tagged value must be a String, Integer, Float, Boolean, or a slice of those types.
- The length of the tag value must be less than 256 characters.
- Nested object values (maps) are not supported for tags.