Adding Sample Data to a Request
You can add other types of metadata to a transaction or span besides tags. The helpers described on this page allow you to overwrite the metadata set by the AppSignal integration or add additional custom data.
Request parameters
By default, the AppSignal integrations track request parameters for web requests in supported libraries. These include query parameters and the POST request body. For background jobs, we store the job arguments in the parameters.
You can set custom parameters on a transaction or span. Modifying the parameters of a transaction will overwrite the data set by the AppSignal instrumentations.
All parameters are filtered by our integrations before being sent to our servers.
See the table below for a list of accepted root values per language. Each nested object can contain values that result in valid JSON (strings, integers, floats, booleans, nulls, etc.).
Language | Accepted root values |
---|---|
Ruby | Arrays, Hashes |
JavaScript | Arrays, Objects |
Elixir | Lists, Maps |
Python | Lists, Maps |
The below code sample shows how to set custom request parameters:
# Values are merged at the top level Appsignal.add_params( :post => { :title => "My title", :body => "Post text." } ) Appsignal.add_params(:user => { :login => "some_name" }) Appsignal.add_params(:user => { :id => 123 }) # Parameters: # { # :post => { :title => "My title", :body => "Post text." }, # :user => { :id => 123 } # }
Appsignal.Span.set_sample_data( Appsignal.Tracer.root_span, "params", %{ post: %{ title: "My new blog post!", body: "Some long blog post text." } } )
import { setParams } from "@appsignal/nodejs"; setParams({ post: { title: "My new blog post!", body: "Some long blog post text.", }, });
from appsignal import set_params set_params({ post: { title: "My new blog post!", body: "Some long blog post text." } })
If the application sets parameters multiple times, the Ruby gem will merge values at the root level. For other integrations, only the last set value is stored.
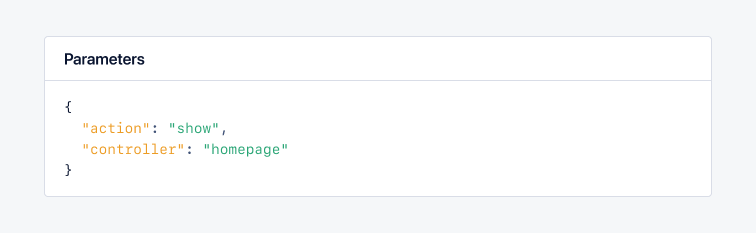
Request headers
By default, the AppSignal integrations track request HTTP headers for web applications in supported libraries.
You can set custom request headers on a transaction or span. Modifying the request headers will overwrite the data set by the AppSignal instrumentations.
All request headers are filtered by our integrations before being sent to our servers.
See the table below for a list of accepted value types for request headers.
Language | Accepted header name | Accepted header value |
---|---|---|
All | String | String |
The below code sample shows how to set custom request headers:
# Call `add_headers` multiple times to set more headers Appsignal.add_headers("REQUEST_METHOD" => "GET", "REQUEST_PATH" => "/some-path") Appsignal.add_headers("PATH_INFO" => "/a-path") Appsignal.add_headers("PATH_INFO" => "/some-path") # Headers: # { # "REQUEST_METHOD" => "GET", # "REQUEST_PATH" => "/some-path", # "PATH_INFO" => "/some-path" # }
Appsignal.Span.set_sample_data( Appsignal.Tracer.root_span, "environment", %{ "request_method" => "GET", "path_info" => "/some-path" } )
import { setHeader } from "@appsignal/nodejs"; setHeader("request_method", "GET"); setHeader("path_info", "/some-path");
from appsignal import set_header set_header("request_method", "GET"); set_header("path_info", "/some-path");
Only the last value for a request header is stored if the application sets a request header multiple times.
For Elixir, if the helper is called multiple times, only the last set of request headers (environment) is stored.
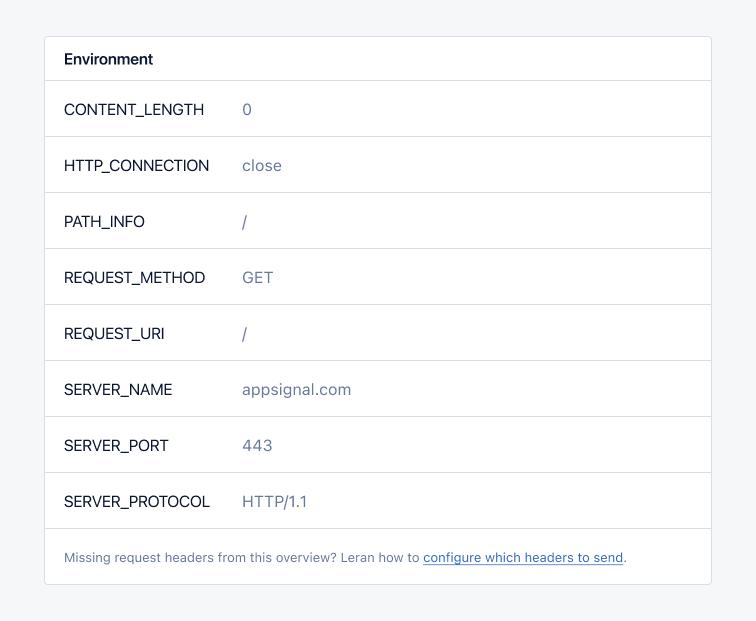
Session data
By default, the AppSignal integrations will track request session data for web applications in supported libraries.
You can set custom request session data on a transaction or span. Modifying the session data will overwrite the data set by the AppSignal instrumentations.
All request session data is filtered by our integrations before being sent to our servers.
See the table below for a list of accepted root values per language. Each nested object can contain values that result in valid JSON (strings, integers, floats, booleans, nulls, etc.).
Language | Accepted root values |
---|---|
Ruby | Arrays, Hashes |
JavaScript | Arrays, Objects |
Elixir | Lists, Maps |
Python | Lists, Maps |
# Call `add_session_data` multiple times to set more session data Appsignal.add_session_data( :user_id => "123", :menu => { :type => "hamburger" } ) Appsignal.add_session_data(:menu => { :state => "closed" }) Appsignal.add_session_data(:menu => { :state => "open" }) # Session data: # { # :user_id => "123", # :menu => { :state => "open" } # }
Appsignal.Span.set_sample_data( Appsignal.Tracer.root_span, "session_data", %{user_id: "123", menu: "open"} )
import { setSessionData } from "@appsignal/nodejs"; setSessionData({ user_id: "123", menu: "open" });
from appsignal import set_session_data set_session_data({"user_id": "123", "menu": "open"})
If the application sets session data multiple times, the Ruby gem will merge values at the root level. For other integrations, only the last set value is stored.
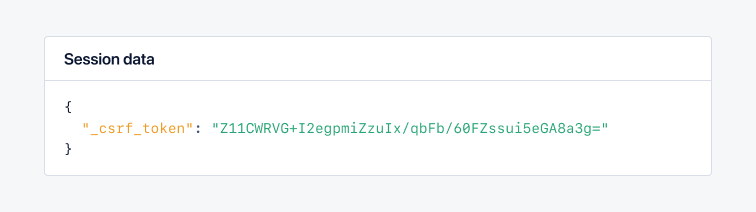
Custom data
You can use custom sample data to set more dynamic values than other types of sample data allow. See the table below for a list of accepted root values per language. Each nested object can contain values that result in valid JSON (strings, integers, floats, booleans, nulls, etc.).
Language | Accepted root values |
---|---|
Ruby | Arrays, Hashes |
JavaScript | Arrays, Objects |
Elixir | Lists, Maps |
Python | Lists, Maps |
It is impossible to filter or search on the data set as custom data. It only provides an additional area in the interface to list more metadata.
When using custom data for nested objects, you can view the object on the Incident Sample page for both Exception and Performance samples formatted as JSON, like in the example below:
# Call `add_custom_data` multiple times to add more custom data # Hash example Appsignal.add_custom_data( :stroopwaffle => { :caramel => true }, :market => { :type => "outdoors" } ) Appsignal.add_custom_data(:market => { :state => :closed }) Appsignal.add_custom_data(:market => { :state => :open }) # Custom data: # { # :stroopwaffle => { :caramel => true }, # :market => { :state => :open } # } # Array example Appsignal.add_custom_data(["value 1"]) Appsignal.add_custom_data(["value 2"]) # Custom data: ["value 1", "value 2"] # Mixed data type example # The Hash and Array data type cannot be mixed at the root level Appsignal.add_custom_data(:market => :open) Appsignal.add_custom_data(["value 1"]) # Custom data: ["value 1"]
# Map Appsignal.Span.set_sample_data( Appsignal.Tracer.root_span, "custom_data", %{ stroopwaffle: %{ caramel: true, origin: "market" } } ) # List Appsignal.Span.set_sample_data( Appsignal.Tracer.root_span, "custom_data", [ "value 1", "value 2" ] )
import { setCustomData } from "@appsignal/nodejs"; // Object setCustomData({ stroopwaffle: { caramel: true, origin: "market", }, }); // Array setCustomData(["value 1", "value 2"]);
from appsignal import set_custom_data # Map set_custom_data({ "stroopwaffle": { "caramel": true, "origin": "market" } }) # Array set_custom_data(["value 1", "value 2"])
If the application sets custom data multiple times, the Ruby gem will merge values at the root level. For other integrations, only the last set value is stored.
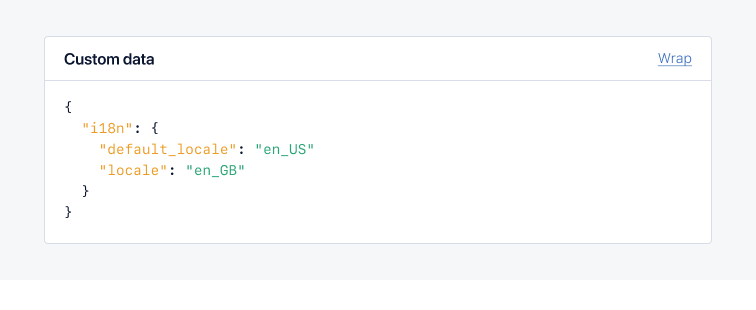